importantThe
CJS
build of Viteโs Node API is no longer supported and will be removed in version 6. As Visual Studio Code extensions do not support ESM, my recommendation is use an alternative bundler such aswebpack
,tsup
, oresbuild
instead of Vite.
A couple of weeks ago, I started looking into the possibility of retrieving the Content Collections from Astro and generating content-types for Front Matter CMS. Eventually, this got me into Vite, and I started to wonder if I could use it to bundle my Visual Studio Code extension to replace the current Webpack setup.
In this article, I will explain how I got it working.
Why Vite?
Vite is a build tool that focuses on speed and simplicity. I want to switch to Vite for the Front Matter CMS extension to speed up the builds for the three bundles I must create (extension and two webview bundles). The webpack setup takes 10-20 seconds before I can start debugging the extension. Vite should be able to do this in a couple of seconds.
Getting started
To start, create a new Visual Studio Code extension project with yo code
. You can choose not to use the webpack setup; we will change the configuration anyway.
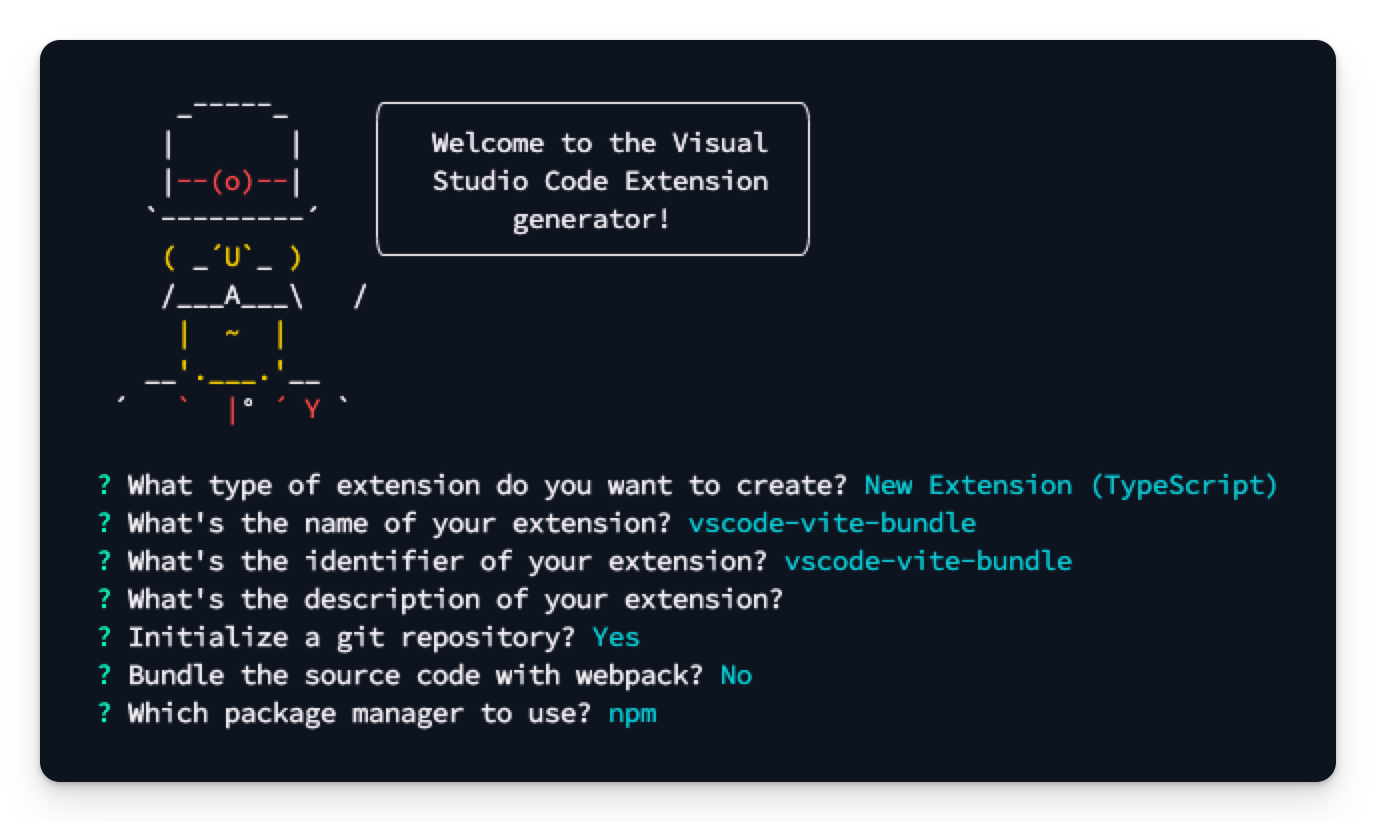
Once the project is created, you can install Vite by running the npm i vite -D
command.
Configuring Vite
The next step is to configure Vite. Create a new file called vite.config.ts
in the root of your project and add the following configuration:
|
|
This configuration will create a CommonJS bundle for the extension, like the current webpack setup. The rollupOptions
exclude the vscode
module from the bundle, as this is already available in the Visual Studio Code environment.
Updating the package.json
In the package.json
file we can update the scripts
section to use Vite.
|
|
Time to test
Now that everything is in place, we can test if the extension still works. Run the npm run compile
command to build the extension.
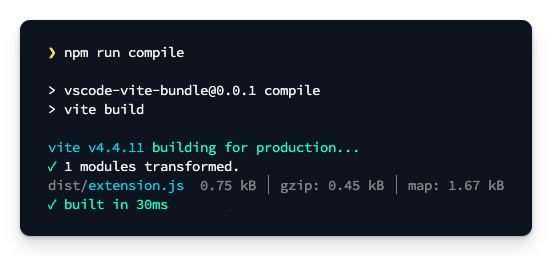
To debug, I typically run the npm run watch
command myself, giving me more flexibility in opening/closing the debugging extension. This flexibility requires a small change in the launch.json
file.
- Open the
launch.json
file - Remove the
preLaunchTask
from the configuration - Save the file
If you applied this change, you can now run npm run watch
and press F5
to debug your extension.
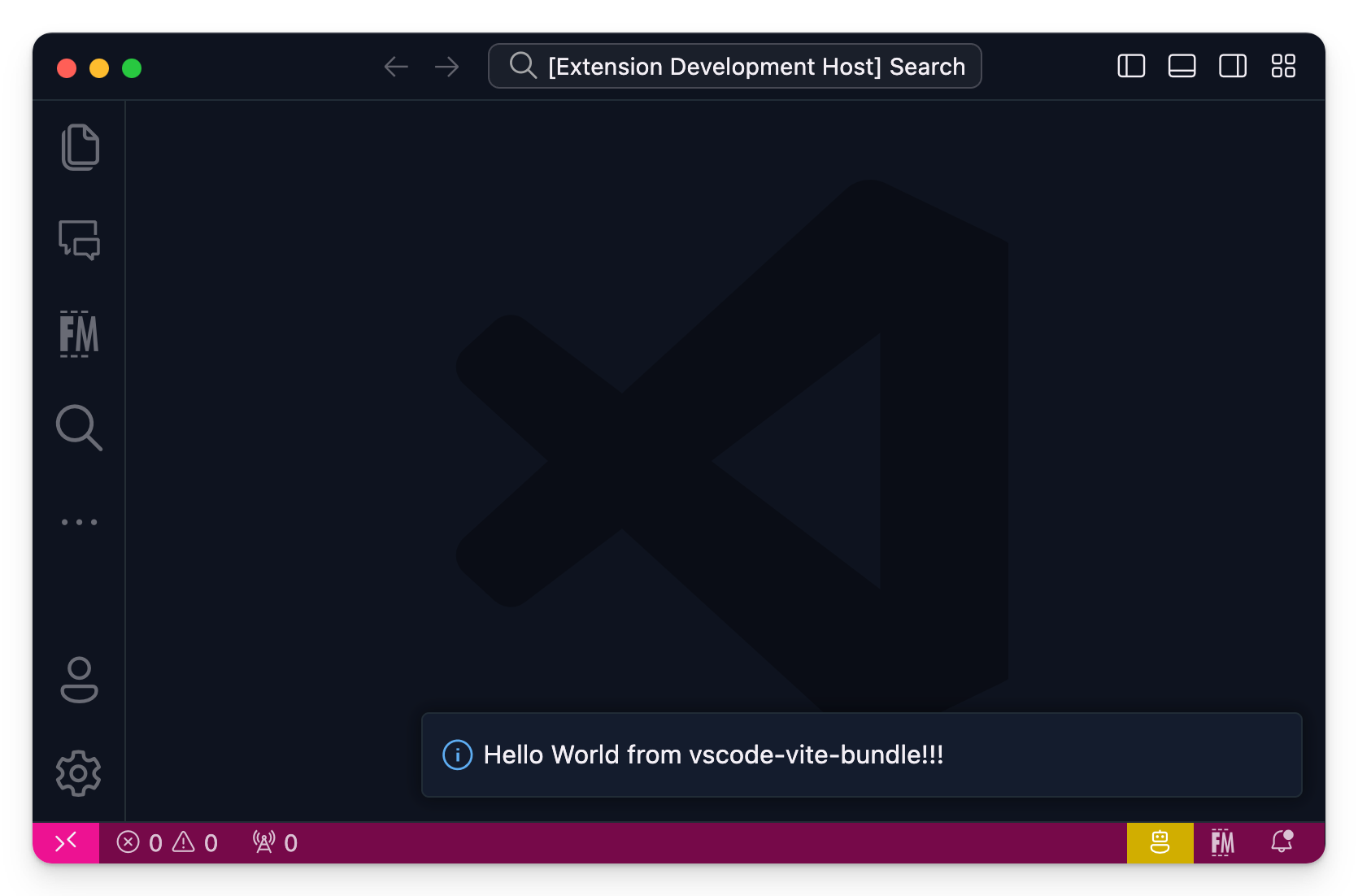