Use command URI in a VSCode webview to open files and links
This post is over a year old, some of this information may be out of date.
If you work with webviews, you may want to open a link to a website or file within the same instance of Visual Studio Code. There are a couple of ways to achieve this, such as posting a message to the extension host or using the command URI option of your webview.
Using the command URI option involves less code and is also the preferred option. Although, you might think, why would I just not use an anchor element and set the HREF attribute to the URL? Well, there is a reason for that, which I will explain in this article.
Why can I not use a hyperlink?
When you want to use an anchor tag with a link to your website for instance, you would typically do it as follows:
<a href="https://elio.dev">elio.dev</a>
It is a quick and easy way, but there is an issue. When you click on the link, it will open immediately in the browser and bypasses the outgoing link protection functionality from Visual Studio Code.
A better approach is to use an internal command like vscode.open
.
The command
The command which can be used to open links/files is vscode.open
and it can be used as follows from your extension:
const devUri = vscode.Uri.parse('https://elio.dev');vscode.commands.executeCommand('vscode.open', devUri);
const fileUri = vscode.Uri.file(join(context.extensionPath, 'readme.md'));vscode.commands.executeCommand('vscode.open', fileUri);
To make use of internal or your commands in a webview, you need to enable the enableCommandUris
option on the webview creation.
The webview configuration
In the code where you create your webview, you can define to enable command URIs as one of the Webview/Panel options.
const panel = vscode.window.createWebviewPanel( 'react-webview', 'React Webview', vscode.ViewColumn.One, { enableScripts: true, retainContextWhenHidden: true, enableCommandUris: true, });
Once you have set this option to true
, you can use anchor tags with HREF attribute set to command:<your command>
.
The webview code
The last step is to write the code to open a link or file. To do this, you will first have to generate a Visual Studio Code URI like object, which looks as follows:
{ scheme: '', path: '', authority: ''}
This object will be used as argument in your command URI.
Opening a link
Here is an example of how you can open a link:
const linkUri = { scheme: 'https', path: '/', authority: 'elio.dev'};
return ( <> <a href={`command:vscode.open?${encodeURIComponent(JSON.stringify(linkUri))}`}>Open link</a> </>)
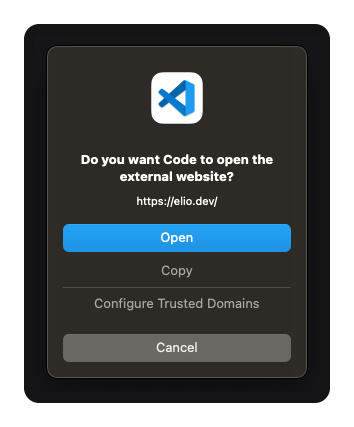
Opening a file
Here is an example of how you can open a file:
const fileUri = { scheme: 'file', path: '<absolute path>/readme.md', authority: ''};
return ( <a href={`command:vscode.open?${encodeURIComponent(JSON.stringify(fileUri))}`}>Open file</a>)
Related articles
#DevHack: Open custom VSCode WebView panel and focus input
#DevHack: How to rename a file from a VSCode extension
In this DevHack we will learn how to rename a file from a vscode extension. If you are looking for a simple appraoch, this will be the one to use.
#DevHack: language-specific settings in a VSCode extension
Get to know how you can set language-specific settings straight from within the code of your Visual Studio Code extension.
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code