In the previous post, I showed how to localize the extension commands/settings and code. In this post, I want to show how to localize the webviews in Visual Studio Code extensions, as this is a bit more complicated and not yet documented.
First, webviews allow you to create fully customizable views for your extensions. I like to use them for Front Matter CMS to display the content/media/… dashboards. The webviews are created with HTML, CSS, and JavaScript. Developing webviews is like web application development running within Visual Studio Code.
To localize these webviews, we can use the @vscode/l10n dependency.
In this article, I will tell you more about how you can get started with localizing your webviews in Visual Studio Code extensions.
Assumptions for your webviews
The contents of this article are written for more advanced webviews where you will use frameworks/libraries like React, Vue, … to render your views.
If you are using the static HTML approach as shown in the documentation sample, you can rely on the vscode.l10n.t()
function to localize your strings.
When you are using a framework/library, you will need to do some extra work to get the localization working in your webviews, and this is what I will explain in this article.
Prerequisites
First of all, follow the steps that were mentioned in the “localizing the strings used in your source files” section of the previous localization article. You must ensure you have configured the l10n
property in your package.json
file.
|
|
Next, we will use the @vscode/l10n dependency. This dependency is used internally by the vscode.l10n.t()
function, but this function is not available in the webview context.
To get started, you will need to install the dependency:
|
|
Localizing your webview
Once the @vscode/l10n
dependency is installed, you can localize your webview’s strings.
infoFor this example, I will make use of the VSCode React Webview Template, which I created to get started with webview development.
Open one of your webview files and import the @vscode/l10n
dependency:
|
|
Next, you must update all your hardcoded strings with the l10n.t()
function.
Example:
|
|
Once all these hardcoded strings are replaced, you can start using the @vscode/l10n-dev
CLI tool to export all your strings to the bundle.l10n.json
file. You can do this by running the following command:
|
|
infoYou can always create your own
bundle.l10n.json
andbundle.l10n.<locale>.json
files, but the tool gives you a good head start.
In my case, when I run the tool, I get the following output:
|
|
importantThe
bundle.l10n.json
is only used for the export and to pass to the translators. To support another language, you must create abundle.l10n.<locale>.json
file with the translated strings.
I translated all the strings to the pseudo-language locale pqs-ploc
for this example. The contents of my bundle.l10n.qps-ploc.json
file look like this:
|
|
Once the strings have been translated, you must implement some logic to get this correctly loaded in your webview. Unfortunately, the @vscode/l10n
dependency will not automatically retrieve the localized strings for you in your subprocesses like the webview.
Retrieve the localization strings for the webview
To stay as close as possible to the logic of how Visual Studio Code handles the localization in extensions, I came up with the following solution.
- From the webview, request the localization strings from the extension host via a
postMessage
call; - The extension host listens for this message and returns the localized strings;
- The webview receives the localized strings and registers them with the
@vscode/l10n
dependency.
To simplify the postMessage
calls, I will use my @estruyf/vscode dependency as it provides a messageHandler
which allows you to async/await
these calls.
Extension host listener for the webview messages
The code for your extension host webview listener looks like this:
|
|
infoThe
vscode.l10n.uri
will only be defined if VS Code is not loaded in the default language and a localization file exists for the current language.
Webview message handler
On the webview side, you will need to implement the following message handler:
|
|
infoOnce the main component loads, it requests the localization content. Once retrieved, it is ready to render its content.
Results
Once you have implemented the logic, you should be able to see the following results:
Default language
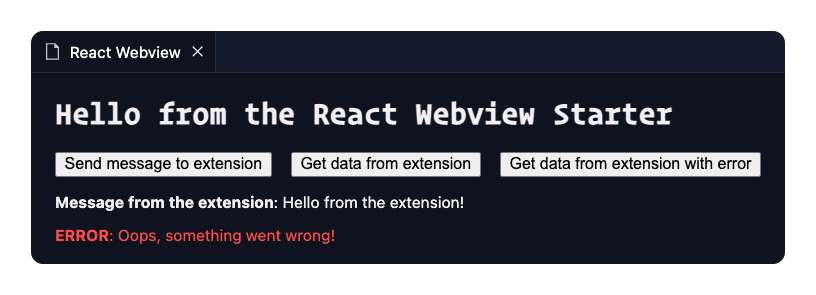
Localized webview (using the pseudo-language)
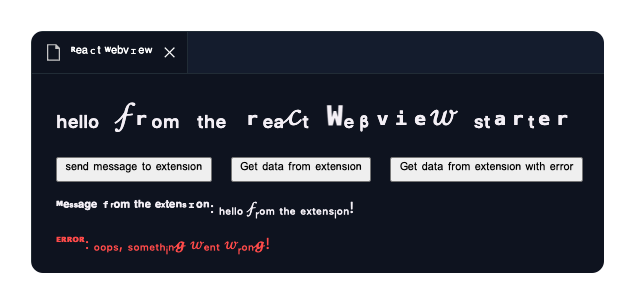
infoThe code for this sample can be found on GitHub.