While creating a custom authentication provider, I found out the standard Microsoft authentication provider in Visual Studio Code can be used with your Azure AD Apps and Tenants.
By default, VS Code supports the github
, github-enterprise
, and microsoft
authentication providers, but little documentation is available, mostly coming from the issues in the VS Code repo.
This article explains how you can use the Microsoft Authentication Provider within your extension.
Using an authentication provider
If you want to use one of the standard authentication providers, all you need to do is get the current session.
|
|
- providerId:
github
,github-enterprise
, ormicrosoft
; - scopes: The permissions scopes to request an access token;
- options: Additional options like specifying if you want to request you to log in if you are signed out.
When the getSession
method returns you an authentication session, you can use the access token on the session object to call the APIs.
The Microsoft authentication provider, as is, is used when you want to sync your settings with a Microsoft account. Although, you cannot use it with your scopes like you can with the github
authentication provider. The Microsoft - Azure AD app used behind the scenes has a predefined permission set configured. You will not be able to change it, but do not worry. There is a way for you to leverage the authentication provider still.
Using the authentication provider with your Azure AD app
When you use the authentication.getSession
method, you must provide the scopes. Luckily, the developers that created the Microsoft Authentication Provider thought about allowing you to provide your Azure AD app. I found the solution for this when I was reading the code of the authentication provider.
Apparently, you have two “special” tokens you can define in the scopes array:
VSCODE_CLIENT_ID:<client-id-value>
VSCODE_TENANT:<tenant-id-value>
Here is an example of how I use it:
|
|
importantThe offline_access scope is very important. You will not get a refresh token if you do not define this. The authentication provider will log you out when reloading your VS Code instance.
Azure AD app configuration
To make this flow work, you need to define the https://vscode.dev/redirect
redirect URI for the Mobile and desktop applications authentication setting on your Azure AD app.
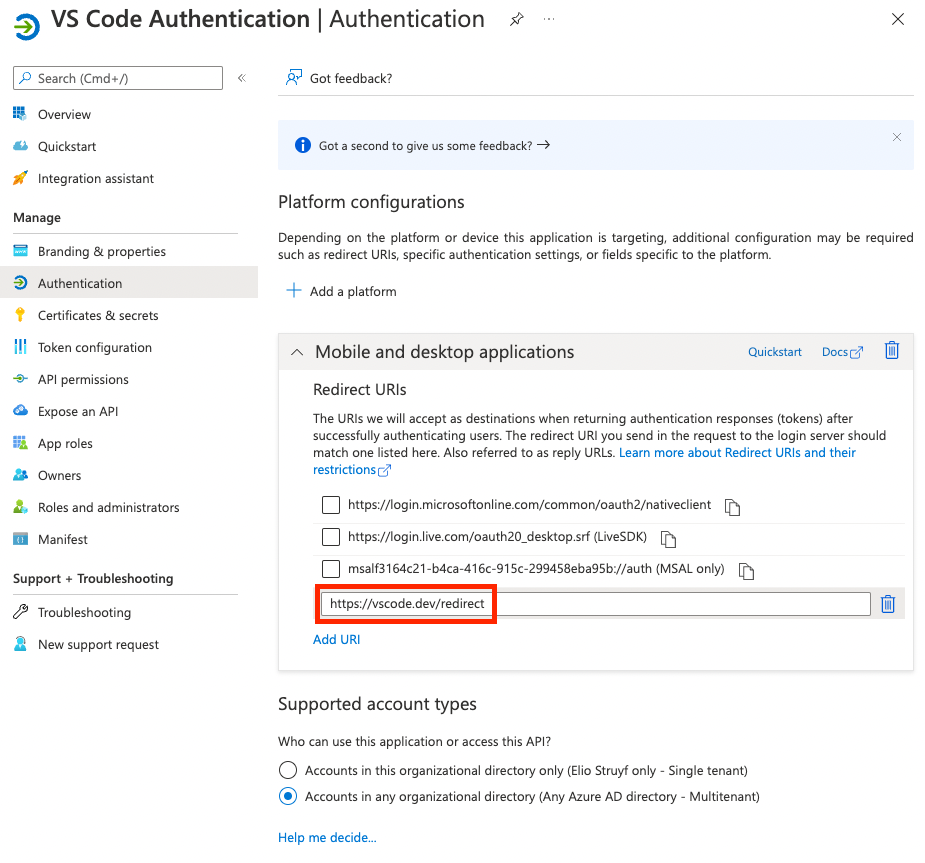
Once this configuration is completed, you can start using the Microsoft Authentication Provider in your extension.