GitHub Actions Job Summaries are a great way to provide more information on your job’s output. This summary is shown in the Actions tab of your repository.
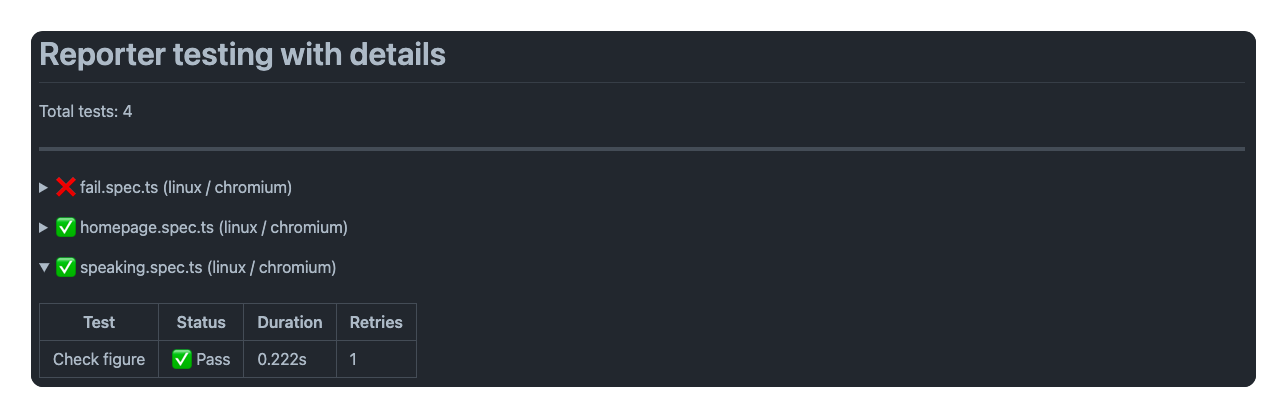
infoYou can read more about it on Supercharging GitHub Actions with Job Summaries
In this post, I’ll explain how you can locally develop and test your GitHub Actions Job Summary outputs using the @actions/core dependency.
infoTesting your summary locally will save you some time, as you don’t have to push your changes to GitHub to see if your summary output is working as expected.
In my case, I used this approach to develop the GitHub Actions Reporter for Playwright. This npm package generates a summary output for your Playwright tests.
How to write a summary output
The easiest way to write a GitHub Actions summary is to use the @actions/core dependency. The @actions/core
dependency provides functions for inputs, outputs, logging, and more.
You can write the job summary using the core.summary
methods. Once completed, you can call the core.summary.write()
method to write the buffer to the Job Summary output on GitHub Actions.
|
|
The downside of this approach is that you have to push your changes to GitHub to see if your summary output is working as expected. When you try to run it locally, it will throw an error saying it is unable to find the GITHUB_STEP_SUMMARY
environment variable.
Testing your summary locally
To test your summary locally, you must set the GITHUB_STEP_SUMMARY
environment variable. This variable defines the file path where the summary output should be written. Locally, we can pass a local file path to this environment variable.
Here is an example of how to set the GITHUB_STEP_SUMMARY
environment variable when running your script locally.
|
|
When you run your script locally, you will see that the summary.html
file is created with the content of your summary output. The generated file contents look like this:
|
|