SharePoint Framework unit-tests with Jest
This post is over a year old, some of this information may be out of date.
Back in April 2017, I wrote about how you could write unit tests for SharePoint Framework components. This was created by a combination of the dependencies provides by SharePoint Framework itself and enzyme (JavaScript Testing utilities for React).
Info: you can read more about writing unit tests for SharePoint Framework components here: Writing unit tests for your SharePoint Framework components.
There are a couple of downsides to this approach:
- Unit tests are slow as the full SPFx build process needs to run every time you want to run your Unit Tests.
- Harder to debug your unit tests as it is tightly coupled in the SharePoint Framework build process. Read more about it here: How to debug your SharePoint Framework unit-tests.
- Integration with other systems and tools is harder to achieve as it requires you to override the existing Karma configuration. Read more about it here: SharePoint Framework code coverage reports for unit-tests.
Let’s decouple our unit-tests from SPFx build process
An easier way to test your solutions would be to decouple your unit-tests process from the SPFx build process. Of course, this is now so easy, but luckily there are some great tools which make is fairly easy. The tool I like to use these days for running my unit-tests is Jest. Jest calls itself a delightful JavaScript testing framework, and I must agree that it is easy to configure. The documentation is great to get you started. This article will show you how to configure Jest in your SharePoint Framework solutions so that you can quickly get started writing your unit-tests.
Let’s get started
As the base of this article, I’ll use the project I created for my first unit-tests article: https://github.com/estruyf/spfx-testing-wp. This project contains a web part with a couple of already defined unit-tests.
To run our tests, we need the following developer dependencies:
- enzyme
- enzyme-adapter-react-15
- jest
- identity-obj-proxy: allows you to test SASS / LESS / CSS imports - https://github.com/keyanzhang/identity-obj-proxy
- react-test-renderer
- react-addons-test-utils
- ts-jest: TypeScript preprocessor with sourcemap support for Jest - https://github.com/kulshekhar/ts-jest
Run the following command to install all at once: npm i enzyme @types/enzyme enzyme-adapter-react-15 @types/enzyme-adapter-react-15 identity-obj-proxy jest @types/jest [email protected] [email protected] ts-jest -D -E
.
Important: use the same versions for react-addons-test-utils and react-test-renderer as the React version defined in the package.json file. At the moment of writing this article, this was version 15.6.2.
Once installed it is time to configure Jest. As we installed ts-jest it allows us to add the Jest configuration to the package.json file. The most basic config is the following:
This is a slightly adapted version of the basic configuration which is shared on the ts-jest documentation. I applied the following changes:
- transform: added ts;
- moduleFileExtensions: removed jsx and node. As most of the time you will work with TS and TSX files;
- moduleNameMapper: configuration for CSS and SCSS files. Otherwise, the unit-tests fail because the test runner does not understand what to do with these files.
In order to run your unit-tests, it might be best to do a change in the scripts property of your package.json file. In the scripts property, you see that test is already defined to gulp test.
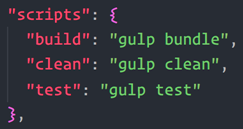
To run Jest, change “gulp test” to jest. That would be all. Once you did this, you can run your test as follows: npm test
.
In many cases, this configuration is already enough, and the output will be as follows:
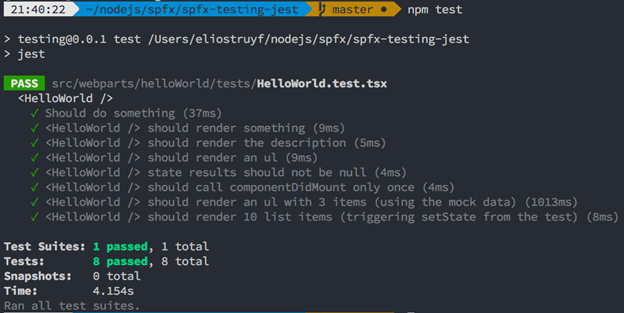
Depending on your usage of SharePoint Framework resources, it might be that you run against the following issue:
Error: Cannot find module ‘resx-strings/en-us.json’ from ‘SPCoreLibraryStrings.resx.js’
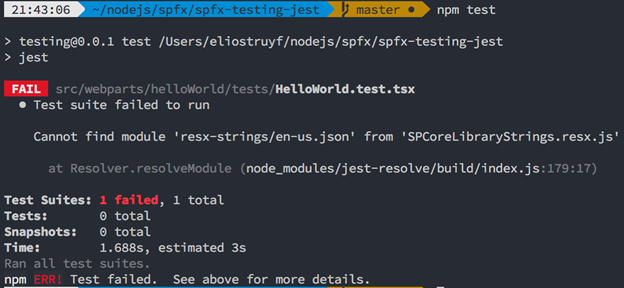
Apparently, the test runner cannot find the en-us.json file. In order to make it work, you can add a mapping for this file in the already defined moduleNameMapper property like this:
When running the test again, test output is the same as before:
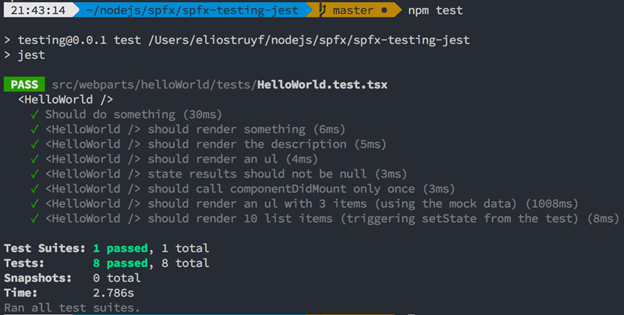
Writing unit-tests
Unit-tests can be written with the use of enzyme. A sample of this is provided in the project. One requirement is that the test files have eighter test or spec in their name.

Info: if you want to reference other test files, you will have to make your changes in the transform property.
What are the benefits of using Jest
In my opinion, the benefits of using Jest in your SharePoint Framework solutions are:
- Unit-tests are fast!
- Easier to test and debug.
- Much easier to extend. It is only a matter of installing the dependencies and applying the configuration. Like for instance tools like Istanbul (Istanbul Jest configuration).
Testing out this sample
If you are interested in testing out this sample project, you can check out the code here: https://github.com/estruyf/spfx-testing-jest.
In the SharePoint SPFx sample repository, you can find another great sample from Velin Georgiev (@VelinGeorgiev): https://github.com/SharePoint/sp-dev-fx-webparts/tree/master/samples/react-jest-testing.
Related articles
Writing unit tests for your SharePoint Framework components
SharePoint Framework code coverage reports for unit-tests
Extend Karma to get better code coverage and test reports for your SharePoint Framework solutions
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code