For a migration from Disqus over to GitHub Discussions, I created a script that processes all comments and replies. One thing I noticed is that I quickly reached the rate limit with my personal access token, which prevents me from using GitHub for a while.
I decided to use a GitHub App to overcome the issue of locking out my account. That way, when the app reaches the rate limit, I can still use GitHub. Although, one thing I just learned was that you need to get the installation token to call the GraphQL API.
In this article, I’ll explain how you can call the GraphQL API from a GitHub App.
App creation
The GitHub Apps page is where you create a new GitHub App.
For calling the GraphQL API, you need to specify the name, and URL, turn off the webhook functionality and provide the required permissions.
Once you have created the app, you can generate a client secret and copy it as you need later.
Generate a new private key and keep the *.pem
file safe.
App installation
On the install app page, install the app to all or the repositories of your choice.
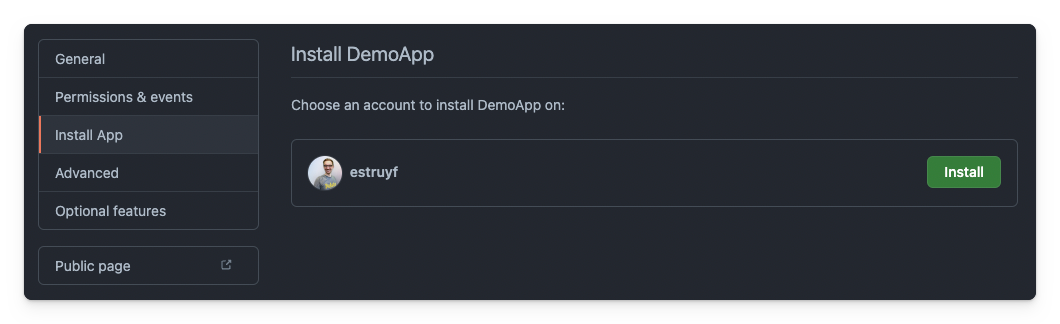
Get an access token
As mentioned, to call the GraphQL API, you must get an installation token. The retrieval of the token comes in two steps:
- Authenticate the GitHub App to get a JWT token;
- Use the JWT token to retrieve the installation ID;
- Use the JWT token to retrieve the installation’ access token.
To get the JWT token, you will need the GitHub app ID, client ID, client secret, and private key. To simplify the code, you can use @octokit/auth-app.
|
|
Once you have the installation’s access token, you can call the GraphQL API.
|
|