Lately, I have been working with the Microsoft’s Dev Proxy, a tool for API simulation, mocking, and testing. One of the things I wanted to try was to use the Dev Proxy in a GitHub Actions workflow so that I could use it in combination with Playwright to test my solutions with mocked APIs.
The Dev Proxy is a .NET Core application that can run on any platform that supports .NET Core, so it works on Windows, Linux, and macOS. I chose to use a macOS virtual machine/runner because, at the time of writing, the Dev Proxy cannot automatically trust the root certificate.
infoCurrently there is an issue open on the Dev Proxy GitHub repository to add support for trusting the root certificate on macOS - Dev Proxy #601.
In this blog post, I will show you how to use the Dev Proxy in your GitHub Actions workflow on a macOS virtual machine.
importantBe aware of that jobs running on Windows and macOS runners that GitHub hosts consume minutes at 2 and 10 times the rate that jobs on Linux runners consume. You can read more about it in the GitHub Actions - Minute multipliers for hosted runners documentation section.
Installing the Dev Proxy
Let us start with installing the Dev Proxy on the macOS virtual machine. We can use the bash script provided in the Dev Proxy documentation for this. To include this into your GitHub Actions workflow, you can use the following step:
|
|
Running the Dev Proxy as a background service
Once installed, you can run the Dev Proxy as a background service by adding an ampersand &
at the end of the command.
infoYou can read more about it in the #DevHack: Running a background service on GitHub Actions article.
Here is what the GitHub Actions step looks like:
|
|
What about HTTPS endpoints?
When you start using the Dev Proxy like this on GitHub Actions, you will encounter issues when calling HTTPS endpoints. In my case, I got the following error when testing it with Playwright:
|
|
These issues are normal because to intercept HTTPS traffic, you must trust the root certificate which gets created by the Dev Proxy. When you run the Dev Proxy on your local machine for the first time, it will prompt you to trust the root certificate.
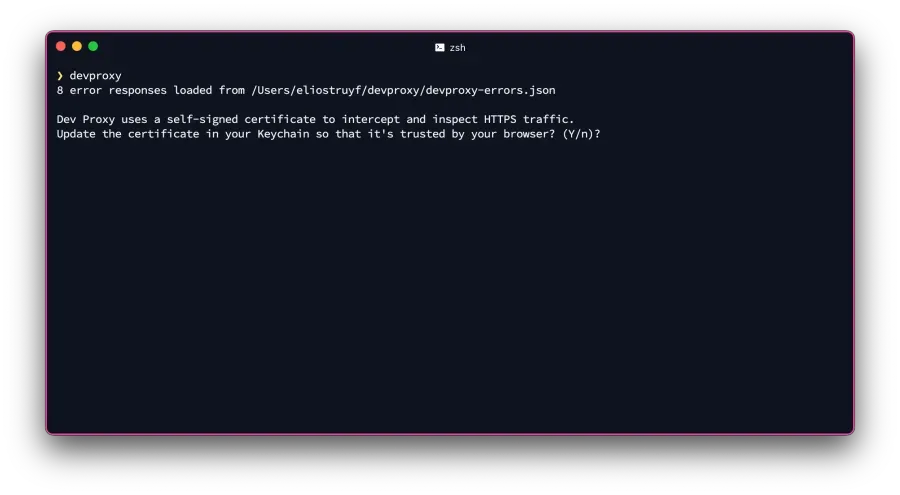
As it runs in a non-interactive mode in GitHub Actions, you cannot trust the certificate that way. To solve this, we will have to include a couple of extra steps in our workflow to trust the root certificate and be able to intercept the HTTPS traffic.
Trusting the root certificate
When the Dev Proxy asks you to trust the root certificate, and you accept it, it uses the trust-cert.sh script to find the certificate and trust it.
My first attempt was to run the trust-cert.sh
script in my GitHub Actions workflow. Unfortunately, this did not work because the security add-trusted-cert
command requires a password to add the certificate to the login keychain.
After some research, I found an article about Trusting Certificates in System Keychain without Prompting. The article explains that you will not be prompted for a password when you add a certificate to the system keychain, and this will be our solution to make it work in our GitHub Actions workflow.
The script to trust the certificate in the system keychain looks as follows:
|
|
This script is similar to the trust-cert.sh script, but instead of adding it to the login keychain, the certificate gets added to the system keychain.
At the end of the script there are three exports which are all optional.
- The
http_proxy
andhttps_proxy
environment variables can be used to set the system proxy settings on the macOS virtual machine. - The
SSL_CERT_FILE
environment variable is required on macOS to make thecurl
commands work with the Dev Proxy.
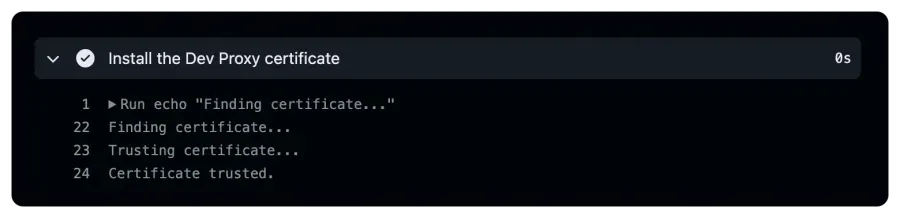
The complete GitHub Actions workflow
Now that we have the certificate trust figured out, we can combine all the steps into a complete GitHub Actions workflow.
|
|
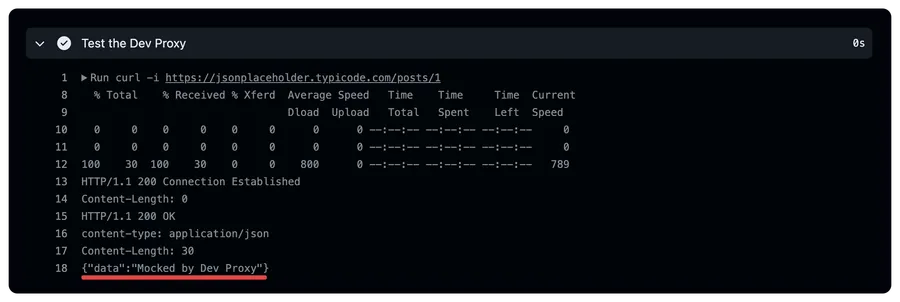
With this setup, you can use the Dev Proxy in your GitHub Actions workflow on a macOS virtual machine. This allows you to use the Dev Proxy in combination with Playwright or any other tool for testing your solutions.
infoTemplates are available on the following GitHub repository.