For one of our internal Office 365 add-ins I wanted to display the user’s profile picture via the Office 365 Unified APIs.
Note: At the moment there is not much documentation about the Unified API and retrieving the user’s profile picture. You could fall back on the documentation about the Outlook User Photo REST API.
In my Office 365 add-in I am displaying the image via JavaScript and the authentication process is done via ADAL.js.
Unified API permissions
To be able to retrieve the user’s profile picture, you first need to add the Sign in and read user profile (Office 365 unified API) permission to your application in Azure Active Directory.

Once this permission is given, you will be able to make your calls.
Unified API call
If you already used the Outlook User Photo REST API you will see that the Unified API call can be done in the same way.
Get photo metadata
If you want to know which user profile picture dimensions are available, you could make use of one of the following endpoints:
Largest available photo
The largest available user picture can be retrieved by calling the following REST endpoint: https://graph.microsoft.com/beta/me/Photo
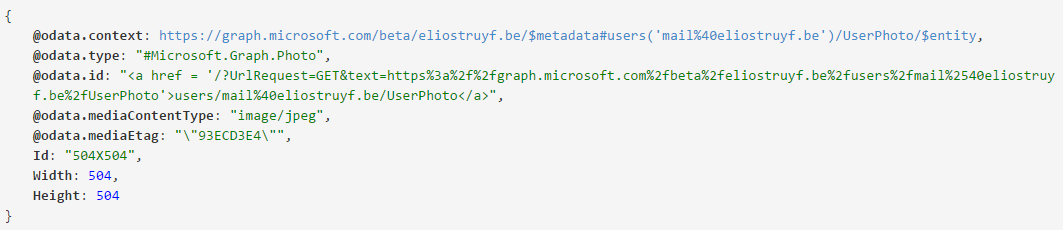
All available photos
If you want to know all the dimensions that are available. You could call the following endpoint: https://graph.microsoft.com/beta/me/Photos
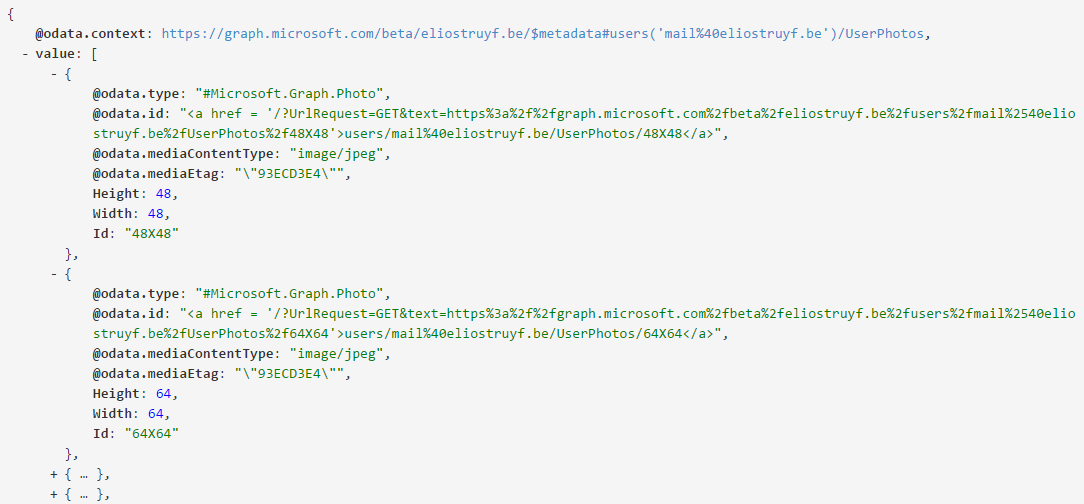
Here is an example how you could call the REST API via JavaScript:
|
|
Retrieve the image (blob) and display it with JavaScript
Once you know which user profile pictures are available, you could retrieve the image via the Unified API.
Get the largest photo
To retrieve the largest user photo, you need to use the following endpoint: https://graph.microsoft.com/beta/me/Photo/$value
Note: notice the $value at the end of the URL, this will make sure you retrieve the image blob instead of the metadata.
Get a specific photo dimension
You are also able to retrieve a photo with a specific dimension. For that you need to use the Id from the photo metadata and append it to your endpoint like this: https://graph.microsoft.com/beta/me/Photos/48X48/$value
Displaying the image blob
Once you know the REST endpoint to use, you need to add a responseType to your XMLHttpRequest in order to retrieve an image blob. Here is my example code:
|
|
In order to read the image blob, the FileReader (more info about FileReader) interface is required. This will be used to convert the blob to a data URL:

You can also use URL.createObjectURL, this method creates a DOMString containing an URL representing the object given in parameter.
Note: More information about this createObjectURL method can be found here: URL.createObjectURL. The code for using this approach looks like this:
|
|
Your element now contains a link to the object in your DOM.

The final output of the two code snippets is the user’s profile image:

Updates
18/09/2015
Updated the post to show the URL.createObjectURL approach. This approach is also explained in an article from Waldek Mastykarz.
3/10/2015
Updated the post with the UserPhoto endpoint that got changed to Photo. More information about this can be found here: Update 3 on Office 365 Unified API.