Take your SharePoint social features to the next level with “I like it” and “Tags” counters: Part 2
This post is over a year old, some of this information may be out of date.
In the previous part I talked about retrieving the number of tags by code. The problem was that the **GetTags **method from the SocialTagManager class, could only retrieve tags from a specific user.
In this part I show you a way to retrieve the all the tags for a specific location/page.
Decompile
My first step was to check the SocialTagManager class to see the code of the GetTags methods. The SocialTagManager class can be found in the Microsoft.Office.Server.UserProfiles.dll.
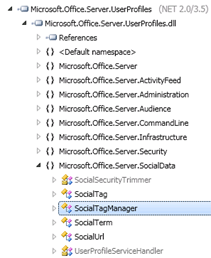
The SocialTagManager class contains 13 GetTags methods, and only four of them are public.
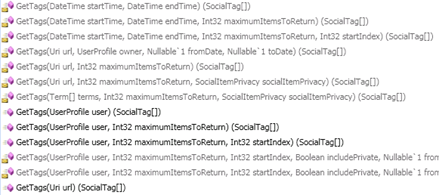
The method that could retrieve all the tags (a maximum of 1000) is the following GetTags(Uri url, Int32 maximumItemsToReturn, SocialItemPrivacy socialItemPrivacy).
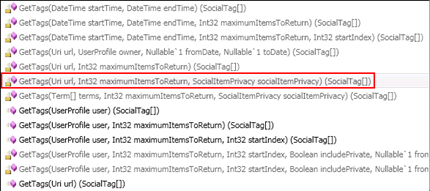
It seems that this method does not use the current user of a specific user to filter the tags. The only problem is that this is an internal method.
The solution is to use reflection, this will allow you to make use of the GetTags(Uri url, Int32 maximumItemsToReturn, SocialItemPrivacy socialItemPrivacy) method.
Reflection
More information about reflection can be found here.
The first thing you need to do is retrieving all the nonpublic methods of your SocialTagManager class. This can be done as follows:
// Retrieve the type of the SocialTagManagervar type = typeof(SocialTagManager);// Get the nonpublic methodsMethodInfo[] methods = type.GetMethods(BindingFlags.NonPublic ' BindingFlags.Instance ' BindingFlags.DeclaredOnly);
Now that you got all the nonpublic methods, you can retrieve the GetTags method. I used the following statement to do this:
MethodInfo method = methods.First(m => m.ToString() == "Microsoft.Office.Server.SocialData.SocialTag[] GetTags(System.Uri, Int32, Microsoft.Office.Server.SocialData.SocialItemPrivacy)");if (method != null){}
The only thing that rests is to invoke the retrieved GetTags method. This can be done like this:
var itemTags = (SocialTag[])method.Invoke(stm, new object[] { uri, 1000, SocialItemPrivacy.PublicOnly });
When you use these code snippets in the console application from part 1, you should now get other results for the items (if you have enough tags, and likes).
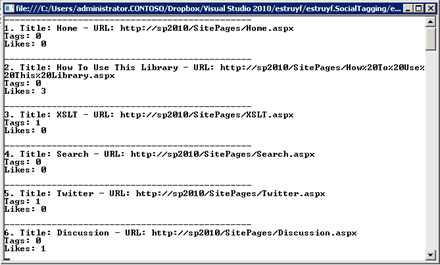
When you compare this result with the first part, you can see that “How To Use This Library” has 2 likes more than in the first part.
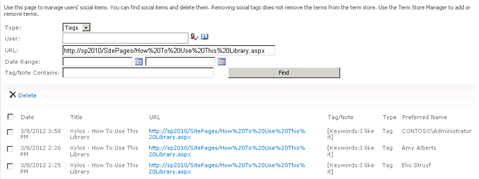
Complete Source Code
Part 3
In the next part I will show you how you can put this in a usercontrol, so that these results can be displayed on all your pages.
Related articles
Take your SharePoint social features to the next level with “I like it” and “Tags” counters: Part 4
Take your SharePoint social features to the next level with “I like it” and “Tags” counters: Part 1
Take your SharePoint social features to the next level with “I like it” and “Tags” counters: Part 3
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code