A couple of weeks ago I wrote a simple Office add-in which just added a custom add-in command. An add-in command only requires that you specify an URL to a page / HTML file to make it work. As it would be overkill to create a website for just that one file, I thought about using an HTTPTrigger Azure Function to return me the necessary HTML for my command.
As it turned out it is quite easy to return the mime type of your choice with an Azure Function. In order to do this, you must specify the content-type header for the object you want to send back as the response. Like for example: HTML is text/html
, XML is text/xml
, text is text/plain
.
Here is an example of an HTTPTrigger function which returns HTML:
|
|
Info: Notice line 9 - 11 where I specified the content-type header.
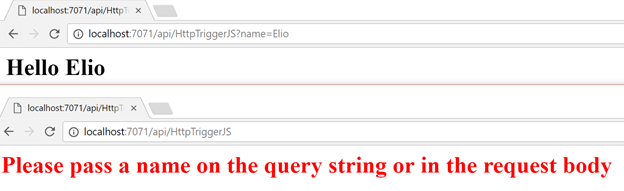