Some time ago I posted an article about how you can check in which context your mail APP has been opened in OWA or the Outlook rich client (read the post here). I needed that solution for a proof of concept mail app.
The mail app is finished, so I wanted to go a step further and I also made the APP work in the other Office applications. An extra functionality I included in the APP was a way to retrieve in which context / rich client the APP was opened. By knowing in which rich client application the Office APP is opened, you can for example match the APP design / colors to that of the rich client application.
At the moment there is no easy way to know in which rich client application your APP has been opened.
Note: this has been written for the Office 1.1 JavaScript API.
The way I found to achieve it is to check the CoercionType which your APP can use. Luckily the rich clients do not use the same CoercionType (more information about the types can be found here).
The following CoercionTypes exist:
- Office.CoercionType.Html: Word
- Office.CoercionType.Matrix: Excel and Word
- Office.CoercionType.Ooxml: Word
- Office.CoercionType.SlideRange: PowerPoint
- Office.CoercionType.Table: Excel and Word
- Office.CoercionType.Text: Excel, PowerPoint, Project, Word Here are some screenshots of the CoercionType object from the various applications:
Word
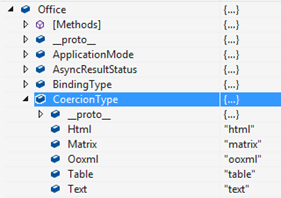
Excel
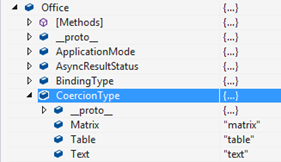
PowerPoint
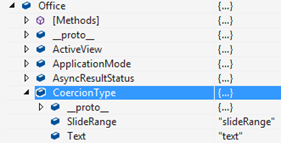
Project
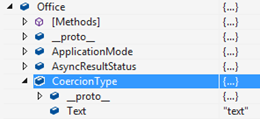
The code
There are a couple of ways to achieve this, here is my approach:
|
|
The code checks which CoercionType is available to use, and sets the background color of the container (you could also do other things, like storing the application name in a variable).
- Ooxml is only available in Word;
- SlideRange is only available in PowerPoint;
- Matrix is available in Excel and Word, but if the APP was opened in Word, the first statement was already true;
- Text this is available in every rich client application, but the last one that remains is Project.
|
|
If you add this code inside your Office.initialize, you get the following outputs:
APP opened in Word
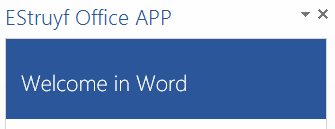
APP opened in Excel
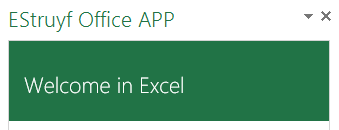
APP opened in PowerPoint
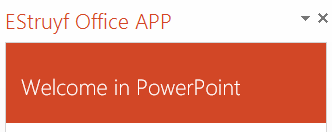
APP opened in Project
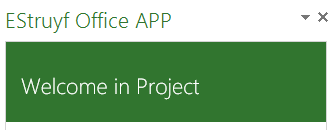