#DevHack: Open custom VSCode WebView panel and focus input
This post is over a year old, some of this information may be out of date.
For the last two weeks, I have been working on my FrontMatter extension for Visual Studio Code. This extension helps others and myself make it easier to manage their static markdown pages’ metadata. Some time ago, I received the question to enhance the tag and category experience. As when you have many tags or categories, it is not optimal to use the selectors/pickers VSCode provides you.
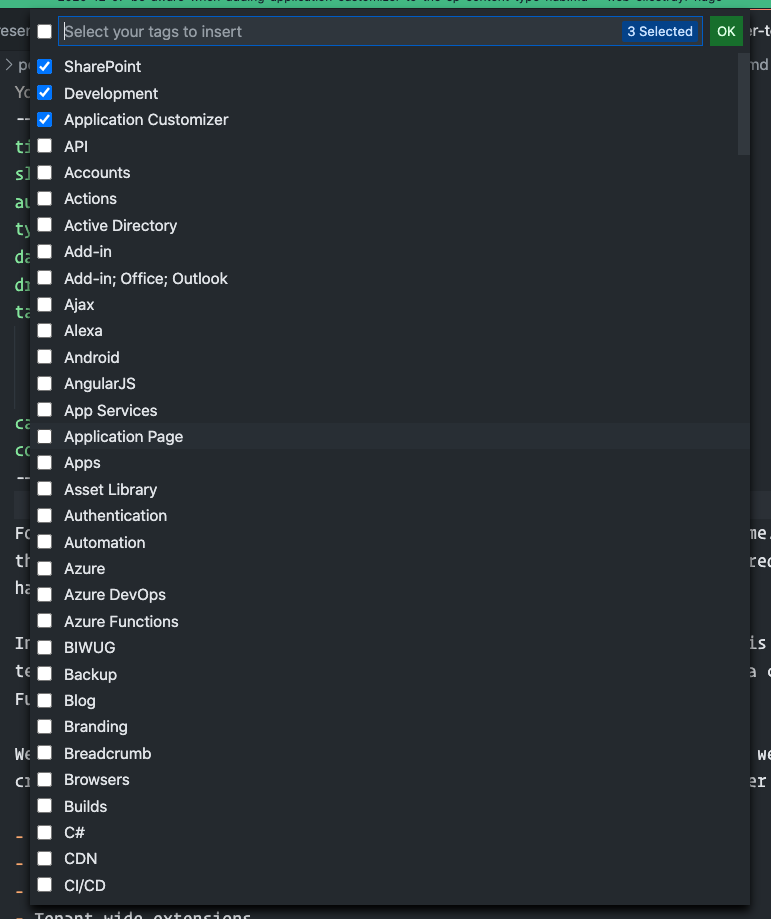
The idea I came up with to improve the experience is to use a custom view panel. From where you can quickly see the SEO recommendations and perform all actions the extension offers. I released this panel functionality in version 1.10.0
.
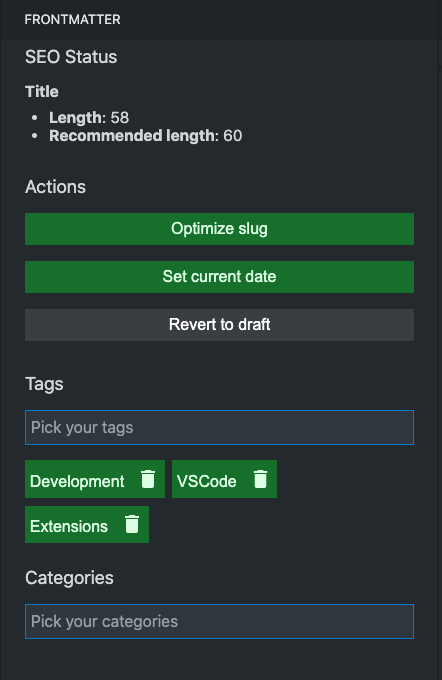
After releasing it, I got positive feedback and the question if I could make it possible to open the panel and focus on the tag/category input field.
The solution is a two-step process:
- Trigger your panel to open
- Post a message to the panel to specify it has to focus on an input
1. Open a panel from your extension
The first step is “easy”, although I was looking at the VSCode API for a long time, which seemed to be the wrong direction. The solution is to use the workbench.view.extension.<your-panel-id>
command.
You can use the following snippet to include it in your extension:
let insertTags = vscode.commands.registerCommand('frontMatter.insertTags', async () => { await vscode.commands.executeCommand('workbench.view.extension.frontmatter-explorer');});
2. Posting message to your panel
Step two is to post a message and needs to perform after opening your extension panel. In my extension, I make use of React in my WebView, and this is not a requirement.
First of all, you need to send a message to your panel to let it know you want to set the focus on the input.
// Post message from your extension to the WebViewthis.panel!.webview.postMessage({ command: "focus" });
In the WebView, where you listen to all these incoming messages, you create the focus listener.
window.addEventListener('message', event => { const message = event.data;
switch (message.command) { case "focus": const elm = document.querySelector('input'); elm!.focus(); break; } });
Once you have these two things in place, you can do something similar to this:
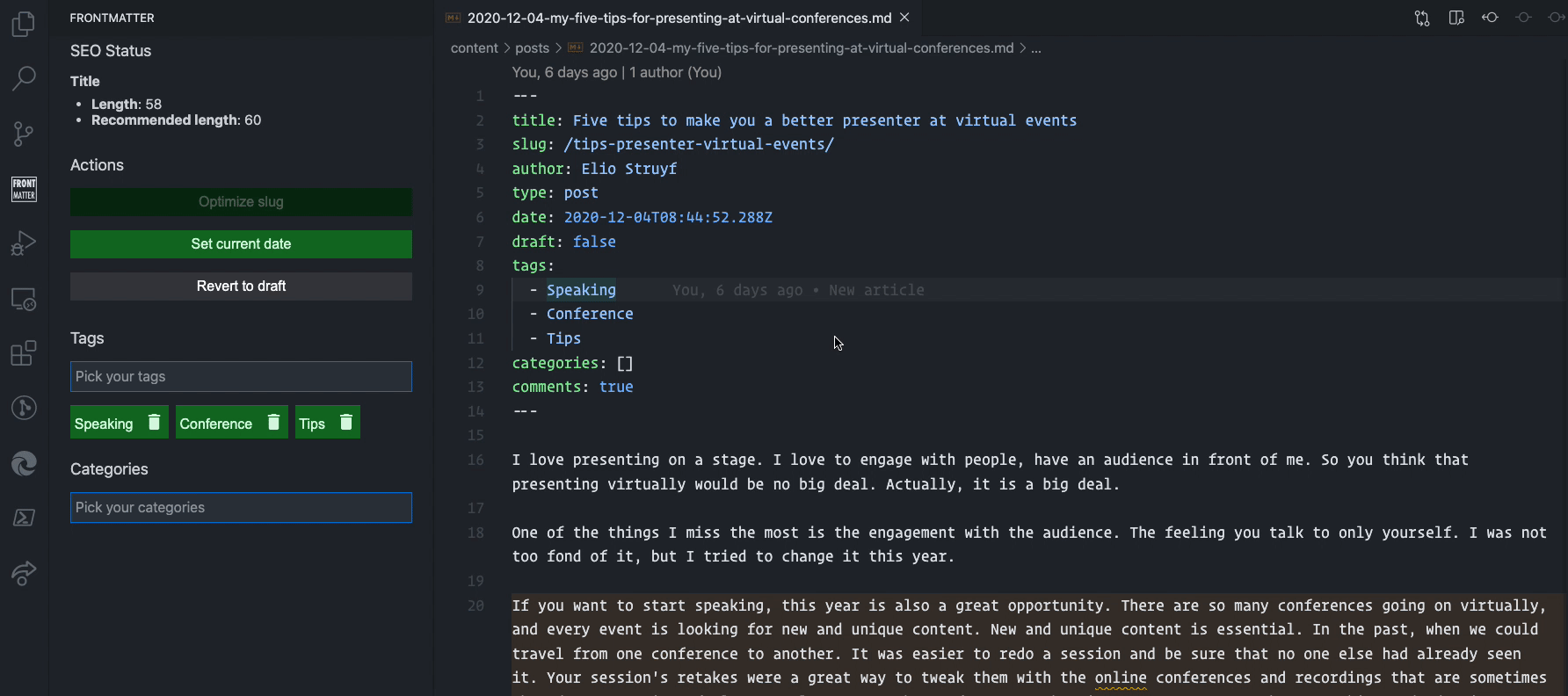
Happy coding
Related articles
#DevHack: How to rename a file from a VSCode extension
In this DevHack we will learn how to rename a file from a vscode extension. If you are looking for a simple appraoch, this will be the one to use.
#DevHack: language-specific settings in a VSCode extension
Get to know how you can set language-specific settings straight from within the code of your Visual Studio Code extension.
#DevHack: check how your VSCode extension is running
This article describes how you can check if your VSCode extension is running in development, production, or test mode.
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code