#DevHack: language-specific settings in a VSCode extension
This post is over a year old, some of this information may be out of date.
When working with Visual Studio Code and different types of languages, you might want to change some settings only for one specific language.
In my case, this was what I wanted to achieve for Markdown. VSCode is my primary editor, which I use for coding and writing this article. I tried to optimize it for writing blog posts, like increase the line height, different font, and more.
The manual approach
You can add Language-specific settings in VSCode, by opening your settings.json
file and specifying a language property name. You do this by using the square brackets []
and writing the language ID you want to target.
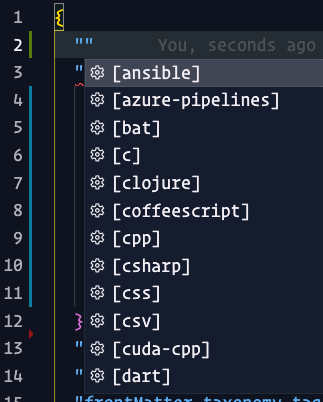
In my case, I went for the following settings for my Markdown-specific project settings:
{ "[markdown]": { "editor.fontFamily": "iA Writer Duo S, monospace", "editor.fontSize": 14, "editor.lineHeight": 26, "editor.wordWrap": "wordWrapColumn", "editor.wordWrapColumn": 64, "editor.lineNumbers": "off", "editor.quickSuggestions": false, "editor.minimap.enabled": false }}
The extension approach
To achieve the same from within your extension, you will have to use the getConfiguration
method. You usually use the getConfiguration
method to retrieve the config settings of your extension by specifying the section
name. When you want to do a language-specific setting, you need to set an empty string for the section
name and specify the languageId
. The looks as follows:
const config = vscode.workspace.getConfiguration("", { languageId: "markdown" });
Once you have the config
object and want to set a new setting value, you use the update
method as follows:
/** * @param section Configuration name. * @param value The new value. * @param configurationTarget If `false` it will update the workspace its settings. * @param overrideInLanguage Specify if you want to update a language-specific setting */config.update("editor.fontSize", 14, false, true);
You can use the get
method as you would normally use it:
const fontSize = config.get("editor.fontSize");
Related articles
#DevHack: Open custom VSCode WebView panel and focus input
#DevHack: How to rename a file from a VSCode extension
In this DevHack we will learn how to rename a file from a vscode extension. If you are looking for a simple appraoch, this will be the one to use.
#DevHack: check how your VSCode extension is running
This article describes how you can check if your VSCode extension is running in development, production, or test mode.
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code