Last week I went to the SharePoint Evolution Conference, which was a great event. At the conference I attended one of Andrew Connell’s sessions about a deep dive into the SharePoint REST API. During Andrew’s session he explained the REST API batching functionality which is at the moment only available in SharePoint Online / Office 365. The batching functionality allows you to batch up a number of HTTP requests to one single request. Andrew Connell already wrote two blog posts about this topic:
- Part 1 - SharePoint REST API Batching - Understanding Batching Requests
- Part 2 - SharePoint REST API Batching - Exploring Batch Requests, Responses and Changesets
The session reminded that I needed to invest some time to optimize the table layout display templates to make use of this REST batching functionality.
Note: You can read more about the table layout display template in this article - Table layout display template with managed property sorting.
If you used or read the article about the table layout display template, you probably know how I used a HTTP request to check if a managed property is sortable or not. The sortable check needs to be performed for every managed property that will be used. Depending on the number of managed properties you want to show with the display template, it results in the same number of HTTP requests. For example: if you configured the CSWP (Content by Search Web Part) to visualize the Path, Title, LastModifiedTime, Created, and Author managed properties it will perform the following requests:

The first two requests returned a 400 error - Invalid parameter: SortList, which means that the corresponding managed property (path and title) are not sortable. By looking at the screenshot above, you could imagine what will happen if you are going to visualize ten managed properties. The REST batching functionality helps you reduce the number of requests that needs to be performed.
If you combine the HTTP requests from the screenshot into one batch it results in the following request:

This request gives the following response:
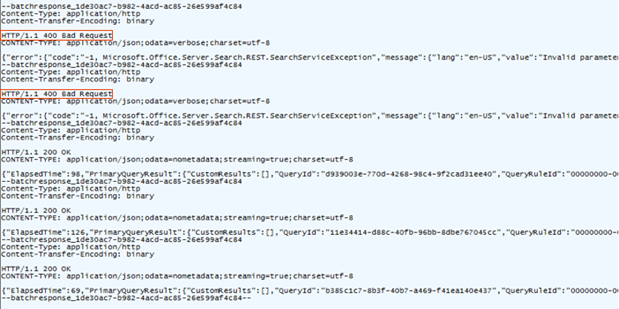
In the screenshot you can see that the response contains the responses for all the combined requests. If you compare this screenshot with the first one, you can see that the first two requests (highlighted in red - path and title) return the same error 400 message - Invalid parameter: SortList.
Updates to the code
To support the batching functionality, the table layout display template codes needed some updates.
First you need to create the batch of requests to check the managed properties.
|
|
Once the batch is created, the request can be done. The code for the batch request looks like this:
|
|
Download
Download the template on SPCSR GitHub Repository: Table layout with sorting template. Make sure that you use the Control_List_Table_Batching.html and Item_List_Table.html display templates.